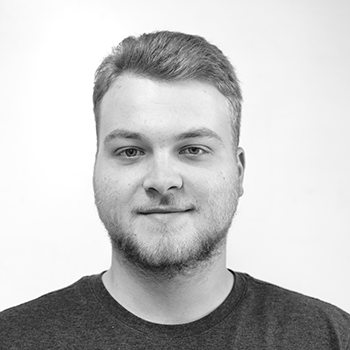
Vincent Bergeron
26 years old // Writing code since 2013 // Seasoned Laravel developer with 7 years of experience // Software developer and Team Lead at @tlmgo
Latest articles
August 29, 2024
From 500 to 404: A Route Model Binding Debug Tale
Today, I had an interesting pairing session with a fellow developer at work. We were going over one of their controllers when I noticed they weren't using Laravel's Route Model Binding in the project. Since it was a relatively small and simple...
ReadJuly 24, 2024
My contribution to laravel/fortify 🎉
Last week, my intern and I were working on a feature that adds logs every time a 2FA-related action occurs. We use Laravel Fortify, and even though Fortify already dispatches a lot of events in various cases, there was no built-in event dispatching when...
ReadJuly 16, 2024
Preventing Transaction-Related Issues in Laravel
Today, I spent a good amount of hours troubleshooting an issue involving database transactions. Thankfully, with the assistance of a teammate, the problem is now resolved. Consider the following example code: In this scenario, we initiate a database...
Read